728x90
나를 위해 기록하는 Flutter 기초
Row로 배치한 요소들을 가로, 세로축을 기준으로 정렬하는 방법
요소 정렬
- mainAxisAlignment
- center
- start
- end
- spaceAround
- spaceBetween
- spaceEvenly
- crossAxisAlignment
- center
- start
- end
- stretch
1. mainAxisAlignment
기준축의 방향으로 정렬
1. center
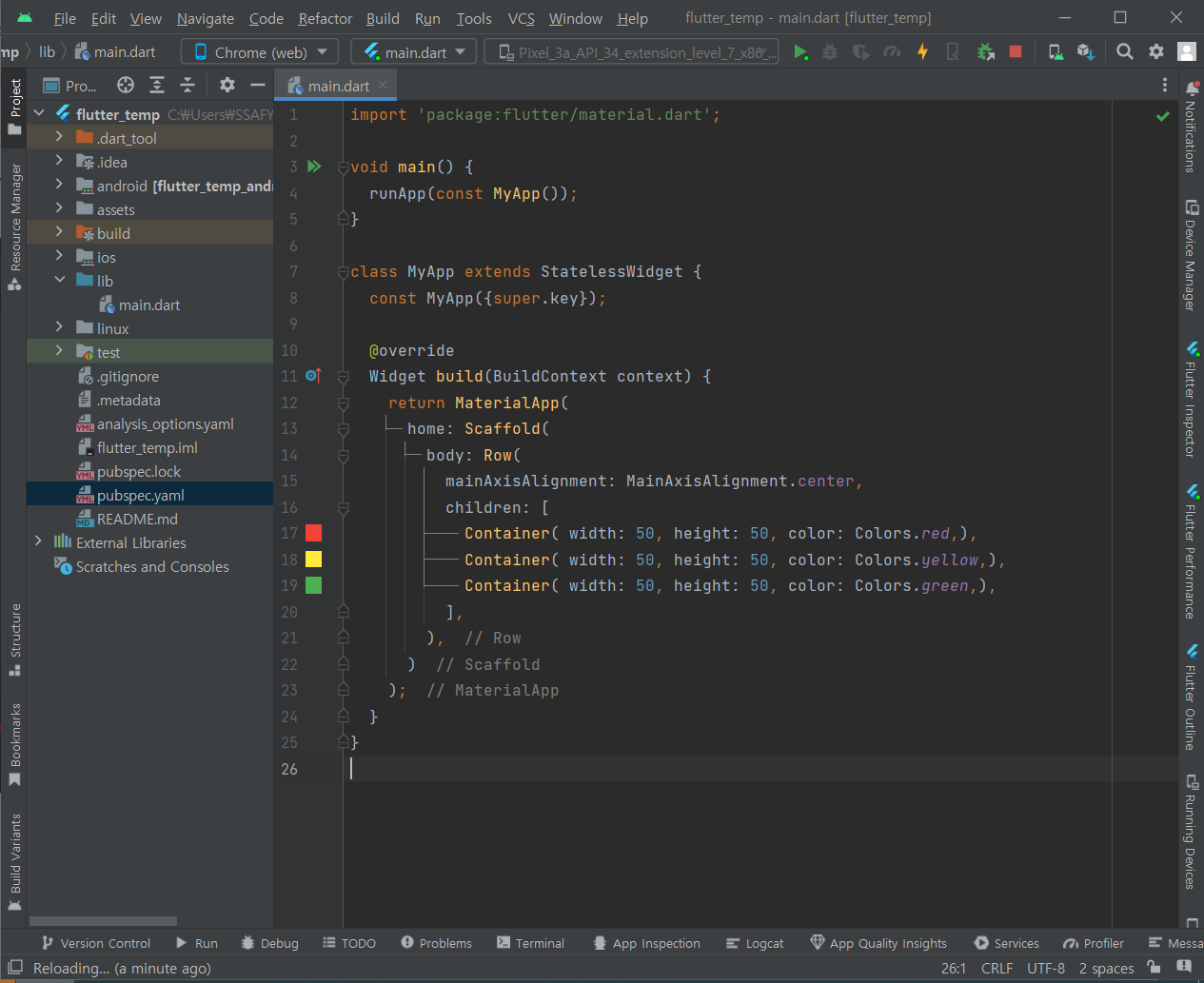
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
);
}
}
- 가운데 정렬
- 요소 사이 빈 공간 없이 모든 요소를 붙여 가운데에 출력

출력 화면 확인
2. start
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Row(
mainAxisAlignment: MainAxisAlignment.start,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
);
}
}
- 왼쪽 정렬
- 요소 사이 빈 공간 없이 모든 요소 붙여 왼쪽 끝에 출력

출력 화면 확인
3. end
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
);
}
}
- 오른쪽 정렬
- 요소 사이 빈 공간 없이 모든 요소를 붙여 오른쪽 끝에 출력
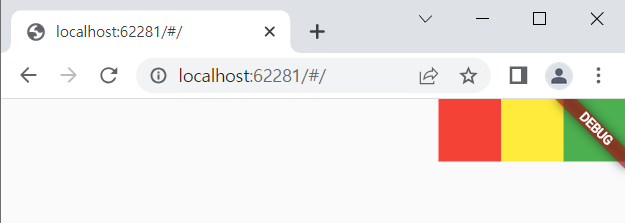
출력 화면 확인
4. spaceAround
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
);
}
}
- 각 요소 사이 공간 생성
- 각 요소의 공간 상에서 각 요소 가운데 정렬
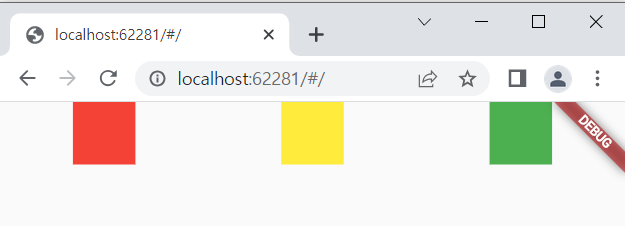
출력 화면 확인
5. spaceBetween
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
);
}
}
- 각 요소 사이 공간 균등 분할
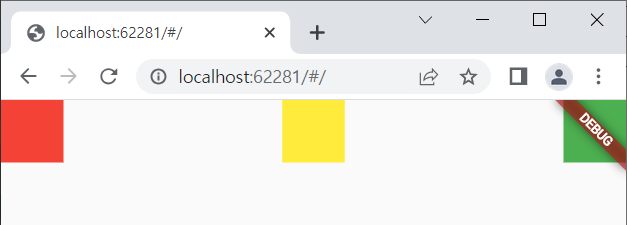
출력 화면 확인
6. spaceEvenly
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
);
}
}
- 각 요소 좌우 공간 균등 분할
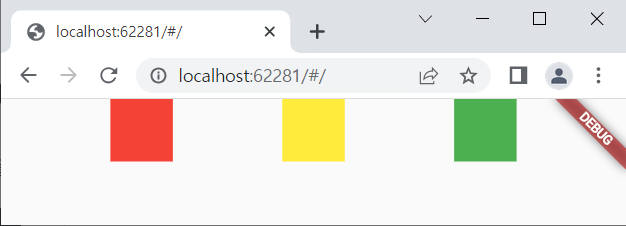
출력 화면 확인
2. crossAxisAlignment
기준축에 대해 수직 방향으로 정렬
1. center
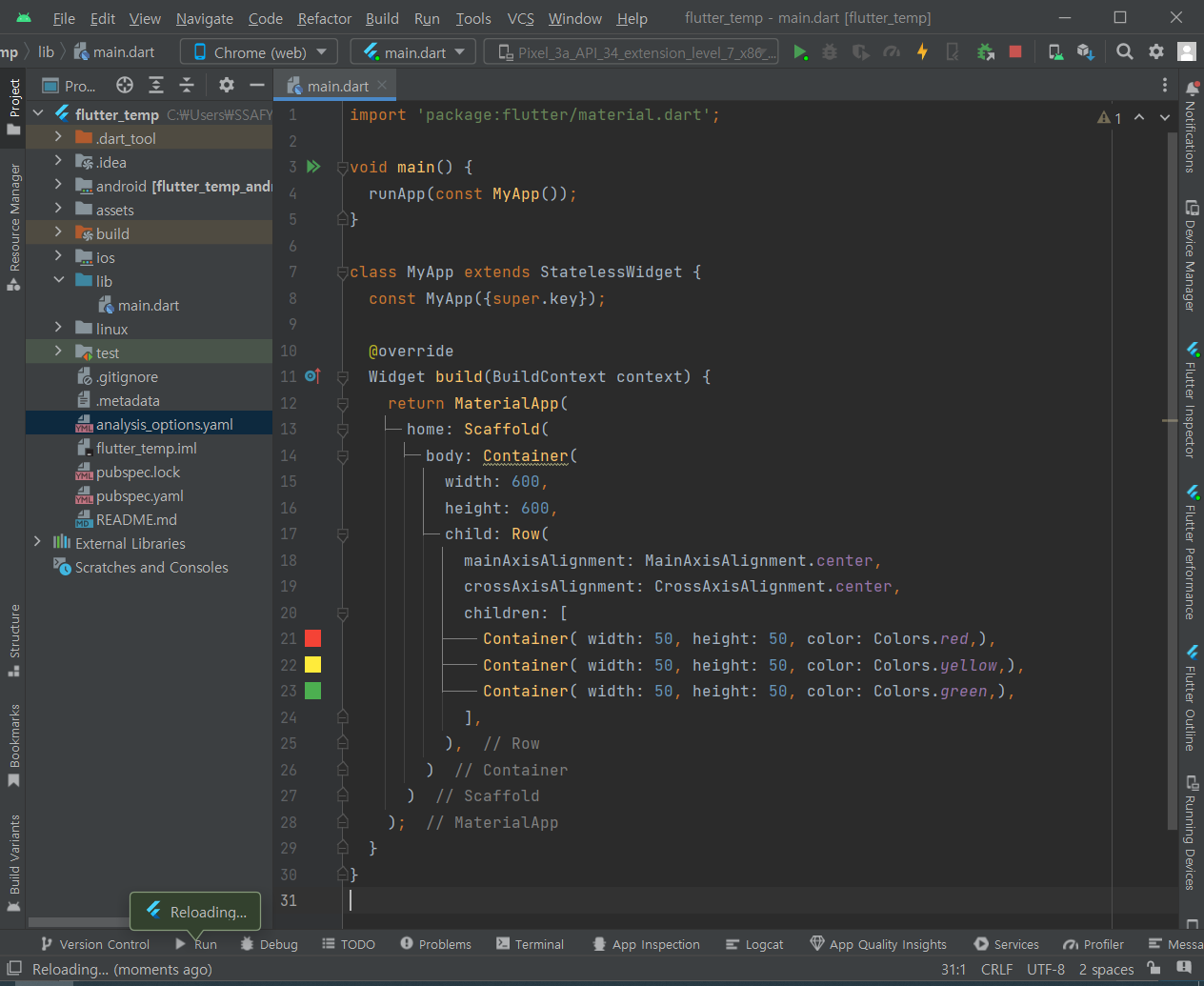
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Container(
width: 600,
height: 600,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
)
);
}
}
- 세로 방향의 가운데 정렬
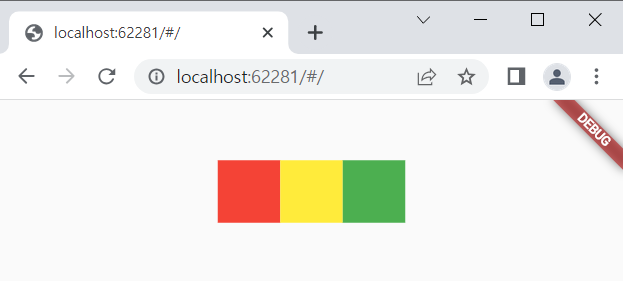
출력 화면 확인
2. start
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Container(
width: 600,
height: 600,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
)
);
}
}
- 세로축 기준으로 왼쪽 시작점 정렬 (위쪽 정렬)
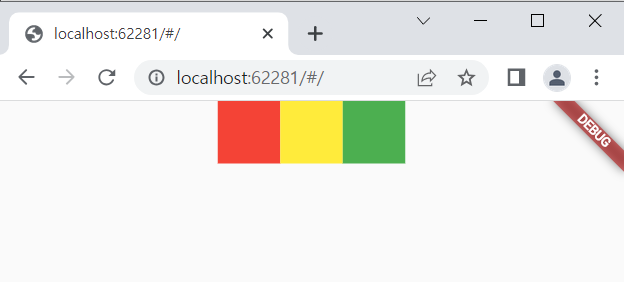
출력 화면 확인
3. end
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Container(
width: 600,
height: 600,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.end,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
)
);
}
}
- 세로축 기준 끝점 정렬 (아래쪽 정렬)
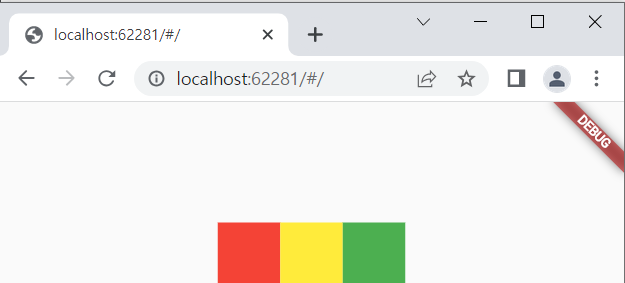
출력 화면 확인
4. stretch
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Container(
width: 600,
height: 600,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container( width: 50, height: 50, color: Colors.red,),
Container( width: 50, height: 50, color: Colors.yellow,),
Container( width: 50, height: 50, color: Colors.green,),
],
),
)
)
);
}
}
- 세로 방향으로 요소가 가득 차도록 배치
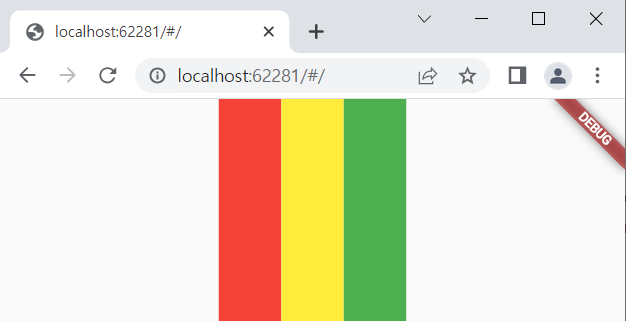
출력 화면 확인
틀린 부분이 있다면 가르쳐주시면 감사하겠습니다.
'Flutter' 카테고리의 다른 글
Flutter 마진(margin), 패딩(padding)으로 여백 만들기 (0) | 2023.07.27 |
---|---|
Flutter Scaffold로 기초 앱 틀 잡기 연습 (0) | 2023.07.25 |
Flutter 가로, 세로 공간 나누기 (0) | 2023.07.21 |
Flutter 위젯 만들기(text, image, icon, box) (0) | 2023.07.19 |
Flutter 프로젝트 시작하기(안드로이드 스튜디오, VS Code) (0) | 2023.07.16 |